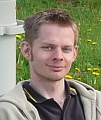
Abhandlung zur Theorie von Gauß-Krüger (zur Information)
Hallo,
Da bin ich froh, ich hätte sonst extra in die Bibliothek der TUM nach München gemusst. Ich selbst bevorzuge die Lösung von Nick Stuifbergen.
Ich habe gerade nachgesehen. Ich hatte es richtig in Erinnerung. Er verweist auf die Methode von Klotz und leitet einen in Fortran implementierten Algorithmus ab, der auch abgedruckt ist.
Sollte auch kein Benchmark-Test sein, aber wer rechnet normalerweise 100.000 Punkte um.
Oh, da kenne ich einige, die ihre alten Daten ins neue UTM-System überführen möchten und dies in einem Rutsch machen.
Doch nicht so komfortabel, aber sicher auch kein Problem.
Naja, die von mir genutzte Klasse findest Du am Ende des Postings. Sie reicht aus für die Grundrechenarten - und mehr als addieren kann der PC ja eh nicht
Für mich persönlich liegt der Mehrwert in der Theorie.
Okay, magst Du Deinen Artikel hier veröffentlichen?
Schöne Grüße
Micha
/********************************************************************** * Copyright (C) by Michael Loesler, http//derletztekick.com * * * * This program is free software; you can redistribute it and/or modify * * it under the terms of the GNU General Public License as published by * * the Free Software Foundation; either version 3 of the License, or * * (at your option) any later version. * * * * This program is distributed in the hope that it will be useful, * * but WITHOUT ANY WARRANTY; without even the implied warranty of * * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * * GNU General Public License for more details. * * * * You should have received a copy of the GNU General Public License * * along with this program; if not, see <http://www.gnu.org/licenses/> * * or write to the * * Free Software Foundation, Inc., * * 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA. * * * **********************************************************************/ package com.derletztekick.tools.geodesy; public class Complex { public final static Complex ONE = new Complex(1.0,0.0); public final static Complex ZERO = new Complex(0.0,0.0); private final double re, im; public Complex(double real) { this(real, 0); } public Complex(double real, double imag) { this.re = real; this.im = imag; } public double abs() { return Math.hypot(this.re, this.im); } public double phase() { return Math.atan2(this.im, this.re); } public Complex plus(Complex b) { return new Complex(this.re + b.re, this.im + b.im); } public Complex minus(Complex b) { return new Complex(this.re - b.re, this.im - b.im); } public Complex times(Complex b) { return new Complex(this.re * b.re - this.im * b.im, this.re * b.im + this.im * b.re); } public Complex divides(Complex b) { return this.times(b.reciprocal()); } public Complex plus(double d) { return this.plus(new Complex(d)); } public Complex minus(double d) { return this.minus(new Complex(d)); } public Complex times(double d) { return this.times(new Complex(d)); } public Complex divides(double d) { return this.divides(new Complex(d)); } public Complex conjugate() { return new Complex(this.re, -this.im); } public Complex reciprocal() { double scale = this.re*this.re + this.im*this.im; return new Complex(re / scale, -this.im / scale); } public double real() { return re; } public double image() { return this.im; } public Complex exp() { return new Complex(Math.exp(this.re) * Math.cos(this.im), Math.exp(this.re) * Math.sin(this.im)); } public Complex sin() { return new Complex(Math.sin(this.re) * Math.cosh(this.im), Math.cos(this.re) * Math.sinh(this.im)); } public Complex cos() { return new Complex(Math.cos(this.re) * Math.cosh(this.im), -Math.sin(this.re) * Math.sinh(this.im)); } public Complex tan() { return this.sin().divides(this.cos()); } public Complex sinh() { return new Complex(Math.sinh(this.re) * Math.cos(this.im), Math.cosh(this.re) * Math.sin(this.im)); } public Complex cosh() { return new Complex(Math.cosh(this.re) * Math.cos(this.im), Math.sinh(this.re) * Math.sin(this.im)); } public Complex tanh() { return this.sinh().divides(this.cosh()); } public Complex coth() { return this.cosh().divides(this.sinh()); } public Complex asin() { double re2 = this.re * this.re; double im2 = this.im * this.im; double t1 = re2 + im2; //double t2 = Math.sqrt( (t1-1.0) * (t1-1.0) + 4.0 * im2 ); double t2 = Math.hypot( (t1-1.0), 2.0 * Math.abs(this.im) ); return new Complex( 0.5*Math.signum(this.re) * Math.acos(t2 - t1), //0.5*Math.signum(this.im) * MathExtension.acosh(t2 + t1), 0.5*Math.signum(this.im) * Math.log((t2 + t1)+Math.sqrt((t2 + t1)*(t2 + t1) - 1.0)) ); } public Complex acos(){ return (new Complex(0.5*Math.PI)).minus(this.asin()); } public Complex log() { return new Complex(Math.log(this.abs()), this.phase()); } public Complex pow(Complex z){ return (z.times(this.log())).exp(); } public Complex pow(double d){ return this.pow(new Complex(d)); } @Override public String toString() { return this.re + " " + (this.im < 0.0 ? "-" : "+") + " " + Math.abs(this.im) + "i"; } public static void main(String args[]) { Complex a = new Complex(3.14, 5.78); System.out.println(a.pow(a)); } }
--
applied-geodesy.org - OpenSource Least-Squares Adjustment Software for Geodetic Sciences
gesamter Thread:
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
16.11.2014, 13:12
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
16.11.2014, 16:26
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
17.11.2014, 09:23
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
17.11.2014, 09:50
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
18.11.2014, 10:37
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
18.11.2014, 10:51
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
18.11.2014, 11:05
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
18.11.2014, 11:58
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
19.11.2014, 11:46
- Abhandlung zur Theorie von Gauß-Krüger - MichaeL, 19.11.2014, 12:20
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
19.11.2014, 13:38
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
20.11.2014, 16:51
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
20.11.2014, 18:00
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
21.11.2014, 15:04
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
21.11.2014, 15:22
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
21.11.2014, 19:45
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
21.11.2014, 19:45
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
21.11.2014, 15:22
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
21.11.2014, 15:04
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
22.11.2014, 11:30
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
24.11.2014, 10:44
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
25.11.2014, 22:53
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
27.11.2014, 10:35
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
27.11.2014, 10:35
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
25.11.2014, 22:53
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
24.11.2014, 10:44
- Abhandlung zur Theorie von Gauß-Krüger -
.seb,
20.11.2014, 18:00
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
20.11.2014, 16:51
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
19.11.2014, 11:46
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
18.11.2014, 11:58
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
18.11.2014, 10:51
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
18.11.2014, 10:37
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
17.11.2014, 09:50
- Abhandlung zur Theorie von Gauß-Krüger -
Rolf,
17.11.2014, 09:23
- Abhandlung zur Theorie von Gauß-Krüger online -
MichaeL,
27.11.2014, 22:58
- Abhandlung zur Theorie von Gauß-Krüger online -
.seb,
27.11.2014, 23:16
- Danke!
- MichaeL, 27.11.2014, 23:20
- Danke!
- Abhandlung zur Theorie von Gauß-Krüger online -
.seb,
27.11.2014, 23:16
- Abhandlung zur Theorie von Gauß-Krüger -
MichaeL,
16.11.2014, 16:26